JavaScript Arrays are list-like objects and it’s prototype has methods to perform traversal and mutation operations. Here we are going to understand the basic operations related to add and remove items from an array. But first let’s understand the basics of arrays and then about the method.
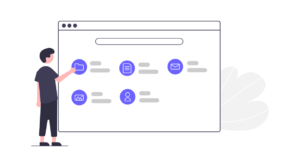
Page Contents
JavaScript Arrays
An array is a collection of elements, which means we can store multiple values in a single variable. In JavaScript, Arrays are represented as an Object.
Let’s understand the need of an array. Consider, you are holding a list of cities and the user has an option to select on the front-end. So to hold those many cities in a single variable, you can use Arrays.
Let’s understand how to create an array.
Syntax:
var arrayName = [element1, element2, ...];
JavaScript Arrays Example:
var cityNames = ['Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore'];
console.log("City names:", cityNames);
//Output City names: [ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
Elements in an array can be accessed using index. Index starts from zero (0) and can be used upto the array length. With that, element ‘Pune’ can be accessed with below code.
console.log("City at 2nd index:", cityNames[2]); //City at 2nd index: Pune
JavaScript Arrays management with push() pop() shift() & unshift()
The JavaScript Array object provides very useful methods for basic operations: push(), pop(), shift() and unshift(). To be precise, these methods belong to the Array object’s prototype property.
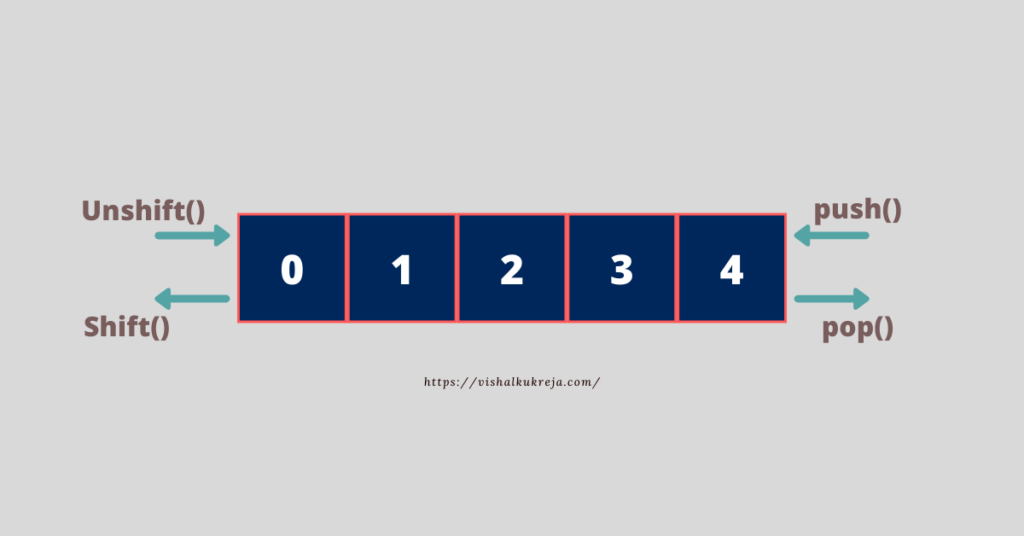
push() – Add an item to the end of an Array
To add a new element to an array, use push() method. The push() method adds one or more elements to the end of an array.
Syntax:
array.push([element1[, ...[, elementN]]])
Example
//add an item to an array
cityNames.push('Jaipur');
console.log("City names:", cityNames);
//Output: City names: [ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore', 'Jaipur' ]
Thus push() added city ‘Jaipur’ at the end of an array.
pop() – Remove an item from the end of an Array
pop() method removes the last element from the end of the array.
Syntax:
array.pop()
Example
//remove an item from an array
cityNames.pop();
console.log("City names:", cityNames);
//Output: City names: [ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
Thus pop() removed city ‘Jaipur’ from end of an array.
shift() – Remove an item from the beginning of an Array
shift() method removes the first element from the beginning of the array.
Syntax:
array.shift()
Example
//Remove an item from the beginning of an Array
cityNames.shift();
console.log("City names:", cityNames);
//Output: City names: [ 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
Thus shift() removed city ‘Mumbai’ from start of an array.
unshift() – Add an item to the beginning of an Array
unshift() method adds one or more elements to the beginning of an array.
Syntax:
array.unshift(element1[, ...[, elementN]])
Example
//Add an item to the beginning of an Array
cityNames.unshift('Chennai', 'Mumbai');
console.log("City names:", cityNames);
//Output: City names: [ 'Chennai', 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
Thus unshift() added city ‘Chennai’, ‘Mumbai’ in the start of an array.
You can find the above code here.
In this article, we learned about the basic of Arrays and Array’s push(), pop(), shift() and unshift() methods. We can leverage these methods to work programmatically with the beginning and end of the array according to our business scenario. If you want to filter elements in an array based on a condition, do checkout this post, and for find() refer this.
For JavaScript array slice() and splice(), do checkout this post.
I hope you enjoyed this post. If you have any question/suggestion, feel free to leave your comments below.
For more details on above methods, you can refer MDN Reference.
Dear reader, a sincere review from you would encourage me to write even more 😉
Stay tuned. Happy Learning.