JavaScript Array slice() and splice() methods are two useful methods to have while performing operations on arrays. These methods are a bit confusing but with little practice or trick, we can remember the functioning of each method. Let’s understand one by one with basic detail and examples.
Page Contents
slice() vs splice()
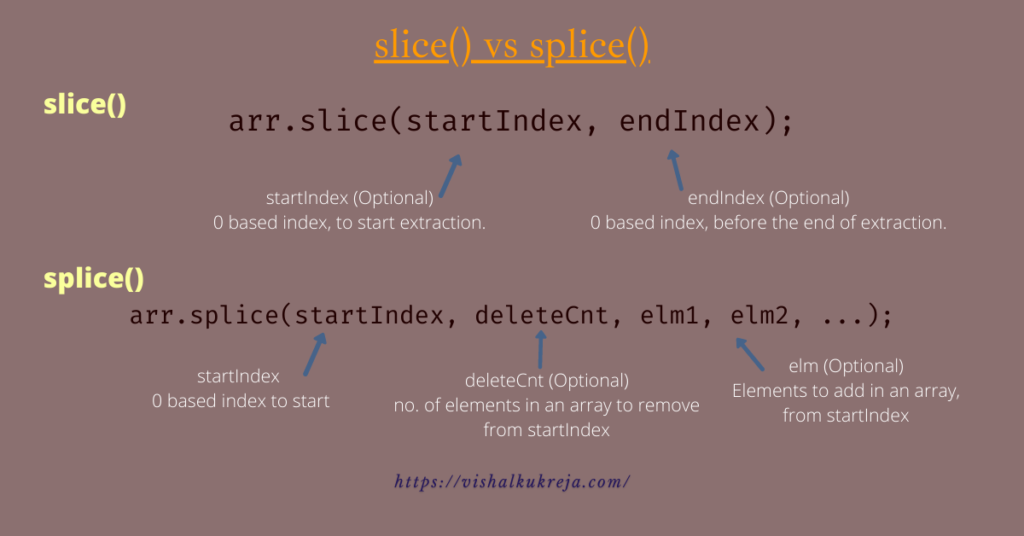
slice() method
It returns a shallow copy of elements from the original array. The slice( ) method copies a mentioned part of an array and returns that part as a new array. It doesn’t change the original array. Examples below shows the various scenarios of using slice() method.
To remember it easily, consider slice as in simple english terms, a piece you cut it from a yummy pizza or a cake. So, you get a new piece from the original one.
Note : Here the original array will not be modified.
Syntax:
arr.slice(startIndex, endIndex);
As shown in an image above, startIndex and endIndex are optional.
Example:
Example below shows the various variants of using slice() method. Like, making a shallow copy of the whole array, extracting required data with different method parameters.
//slice() example
var cityNames = ['Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore'];
console.log("City names:", cityNames);
// Output : City names: [ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
var newCities = cityNames.slice(); // gets all - shallow copy
console.log(newCities); //[ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
var newTwoCities = cityNames.slice(1, 3); // start from 1st index and before 3rd
console.log(newTwoCities); //[ 'Delhi', 'Pune' ]
var remainingCities = cityNames.slice(1); // start from 1st index and extract rest
console.log(remainingCities); //[ 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
// If start is greater than the index or negative, an empty array is returned.
var otherCities = cityNames.slice(-1, 3); //cityNames.slice(5, 4);
console.log(otherCities); // []
var initialCities = cityNames.slice(0, -2); //extract from the start/remove from end
console.log(initialCities); // [ 'Mumbai', 'Delhi', 'Pune' ]
Refer the above code example here.
splice() method
splice() method’s main purpose is modification in an existing array. It changes the contents of an array by removing/replacing its elements or adding new ones. Examples below shows various scenarios of using splice() method. So similarly, with splice() the contents of an array gets changed.
To remember splice() easily, know its basic meaning is to join or connect (a rope or ropes) by interweaving the strands at the ends.
Note : Here the original array will be modified.
Syntax:
arr.splice(startIndex, deleteCnt, elm1, elm2, ...);
As shown in an image above, startIndex is mandate here, deleteCnt and elements are optional. If we provide the negative value in startIndex, it will begin that many elements from the end of an array.
Example
//splice() example
var cityNames = ['Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore'];
console.log("City names:", cityNames);
// Output : City names: [ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
cityNames.splice(1); // if delete count is omitted, from startIndex, all items will be deleted
console.log(cityNames); //[ 'Mumbai' ]
//Add new elements
cityNames = ['Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore'];
cityNames.splice(3, 0, 'Thane'); //delete count is 0, add city Thane @ 3rd index
console.log(cityNames); //[ 'Mumbai', 'Delhi', 'Pune', 'Thane', 'Ahmadabad', 'Indore' ]
cityNames.splice(3, 1,); //delete 1 @ 3rd start index
console.log(cityNames); //[ 'Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore' ]
cityNames.splice(3, 1, 'Surat'); //delete 1 @ 3rd start index and add new element there
console.log(cityNames); //[ 'Mumbai', 'Delhi', 'Pune', 'Surat', 'Indore' ]
cityNames.splice(-1, 1); //delete 1 from start index -1 i.e. from end
console.log(cityNames); //[ 'Mumbai', 'Delhi', 'Pune', 'Surat' ]
cityNames = ['Mumbai', 'Delhi', 'Pune', 'Ahmadabad', 'Indore'];
cityNames.splice(-3); // start index -3 i.e. from end, delete all
console.log(cityNames); //[ 'Mumbai', 'Delhi' ]
Refer the above code example here.
In this article, we learned about the basic slice() and splice() methods. We can leverage these methods to work programmatically with the beginning and end of the array according to our business scenario. If you want to filter elements in an array based on a condition, do checkout this post and this to find().
For modification of arrays using push() and pop() methods, do checkout this.
I hope you enjoyed this post. If you have any question/suggestion, feel free to leave your comments below.
For more details on slice() & splice(), refer MDN Reference.
Dear reader, a sincere review from you would encourage me to write even more 😉
Stay tuned. Happy Learning.